prompt mine App
Find, Create & Share AI Magic
Donut Drop Game Creation
This outlines the creation of "Donut Drop," a simple but engaging browser-based game using JavaScript, HTML, and CSS. The player controls a basket at the bottom of the screen, catching falling donuts to score points. Missing donuts decreases health.
1. HTML Structure (index.html):
html
<!DOCTYPE html>
<html>
<head>
<title>Donut Drop</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<canvas id="gameCanvas" width="800" height="600"></canvas>
<script src="script.js"></script>
</body>
</html>
2. CSS Styling (style.css):
css
gameCanvas {
background-color: lightblue;
display: block;
margin: 0 auto;
}
body {
margin: 0;
overflow: hidden; / Hide scrollbars /
}
3. JavaScript Logic (script.js):
javascript
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
let basketX = canvas.width / 2 - 50; // Basket position
const basketY = canvas.height - 50;
const basketWidth = 100;
const basketHeight = 40;
const basketSpeed = 7;
let donuts = [];
let score = 0;
let health = 3; // Number of lives
// Images (replace with your own donut and basket images)
const donutImage = new Image();
donutImage.src = 'donut.png'; // Make sure this file exists
const basketImage = new Image();
basketImage.src = 'basket.png'; // Make sure this file exists
function createDonut() {
donuts.push({
x: Math.random() (canvas.width - 30),
y: 0,
speed: Math.random() 2 + 1,
width: 30,
height: 30
});
}
function drawBasket() {
ctx.drawImage(basketImage, basketX, basketY, basketWidth, basketHeight);
}
function drawDonuts() {
for (let i = 0; i < donuts.length; i++) {
ctx.drawImage(donutImage, donuts[i].x, donuts[i].y, donuts[i].width, donuts[i].height);
}
}
function updateDonuts() {
for (let i = 0; i < donuts.length; i++) {
donuts[i].y += donuts[i].speed;
// Collision detection
if (donuts[i].y + donuts[i].height > basketY &&
donuts[i].x + donuts[i].width > basketX &&
donuts[i].x < basketX + basketWidth) {
donuts.splice(i, 1);
score++;
createDonut(); // Create a new donut immediately
} else if (donuts[i].y > canvas.height) {
donuts.splice(i, 1);
health--;
createDonut(); //Create a new donut immediately
if (health <= 0) {
// Game Over
clearInterval(gameInterval);
alert("Game Over! Your score: " + score);
}
}
}
}
function drawScore() {
ctx.font = "20px Arial";
ctx.fillStyle = "black";
ctx.fillText("Score: " + score, 10, 20);
}
function drawHealth() {
ctx.font = "20px Arial";
ctx.fillStyle = "red";
ctx.fillText("Health: " + health, canvas.width - 100, 20);
}
// Input Handling
document.addEventListener('keydown', function(event) {
if (event.key === 'ArrowLeft' && basketX > 0) {
basketX -= basketSpeed;
} else if (event.key === 'ArrowRight' && basketX < canvas.width - basketWidth) {
basketX += basketSpeed;
}
});
// Game Loop
function gameLoop() {
ctx.clearRect(0, 0, canvas.width, canvas.height); // Clear canvas
drawBasket();
drawDonuts();
updateDonuts();
drawScore();
drawHealth();
}
// Initialize game
createDonut(); // Create the first donut
const gameInterval = setInterval(gameLoop, 20); // 50 FPS
4. Image Assets:
You will need donut.png and basket.png files. Create these using an image editor, or find suitable images online. Place them in the same directory as your HTML, CSS, and JavaScript files.
Explanation:
HTML: Sets up the canvas element where the game is drawn and links the CSS and JavaScript files.
CSS: Styles the canvas for basic presentation.
JavaScript:
Handles game logic: creating donuts, moving the basket, collision detection, scoring, and health.
Uses setInterval to create a game loop that updates the game state and redraws the screen repeatedly.
Listens for keyboard input (left and right arrow keys) to control the basket.
The createDonut() function creates new donut objects with random horizontal positions and speeds.
Collision detection checks if a donut intersects with the basket.
Game Over is triggered when health reaches 0.
To Run the Game:
1. Save the HTML, CSS, and JavaScript code into separate files (index.html, style.css, script.js).
2. Create or download donut.png and basket.png and save them in the same directory.
3. Open index.html in your web browser.
Possible Enhancements:
Difficulty Scaling: Increase donut speed or spawn rate over time.
Different Donut Types: Add donuts with different point values or effects.
Power-Ups: Implement power-ups that temporarily increase basket size or slow down donuts.
Sound Effects: Add sound effects for catching donuts and losing health.
Graphical Improvements: Use more detailed images and animations.
Mobile Support: Adapt the game for touch controls.
Score Storage: Implement local storage to save high scores.
Levels: Divide the game into levels with increasing difficulty.
Menu Screen: Add a start menu and a game over screen.
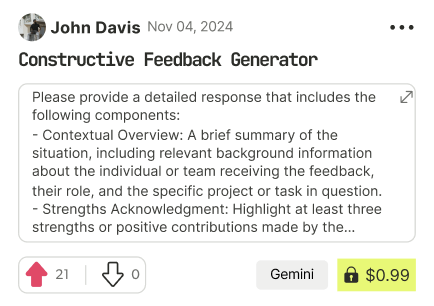
Find Powerful AI Prompts
Discover, create, and customize prompts with different models, from ChatGPT to Gemini in seconds
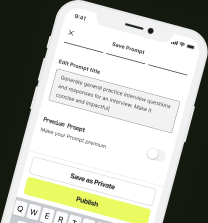
Simple Yet Powerful
Start with an idea and use expert prompts to bring your vision to life!